We’re excited to announce Replit Object Storage, a fast and durable way to persist files and other unstructured data. Object Storage ensures your data is resilient against data loss and usable across the Replit workspace and Deployments. It handles any number of processes reading and writing simultaneously, enabling your application to seamlessly add compute power and scale to handle extra requests.
Our goal with Object Storage was to deliver a solution that enables you to start reading and writing files from your application as quickly as possible. We’ve designed the system to require minimal configuration and work out-of-the-box in both the workspace and Deployments.
Object Storage excels at storing large amounts of unstructured data (think lots of files with varying shapes and sizes). It’s ideal for:
- Storing media assets such as images
- Persisting files uploaded by users such as PDF documents
- Flexible data storage that doesn’t require configuring a database such as CSV and JSON files
Interacting with Object Storage is simple. For example, the program below is a server that uploads and downloads images:
import io
from flask import Flask, request, send_file
from replit.object_storage import Client
# Setup the server and the client for interacting with Object Storage
app = Flask(__name__)
client = Client()
# API for uploading images
@app.route('/upload/<image_name>', methods=['POST'])
def upload_image(image_name):
# Read the uploaded image and upload it to Object Storage
client.upload_from_bytes(image_name, request.get_data())
return "Success"
# API for downloading images
@app.route('/download/<image_name>', methods=['GET'])
def download(image_name):
# Download the image from Object Storage
image = client.download_as_bytes(image_name)
# Format the API response and send the image back to the requester
return send_file(io.BytesIO(image),
as_attachment=True,
download_name=image_name)
Managing application data from the workspace: Object Storage pane
You’ll notice a new tool pane in the workspace: Object Storage. When you open the pane for the first time in a Repl, you’ll be prompted to create a Bucket. Buckets are containers for storing data; everything you store in Object Storage must be stored inside a Bucket.
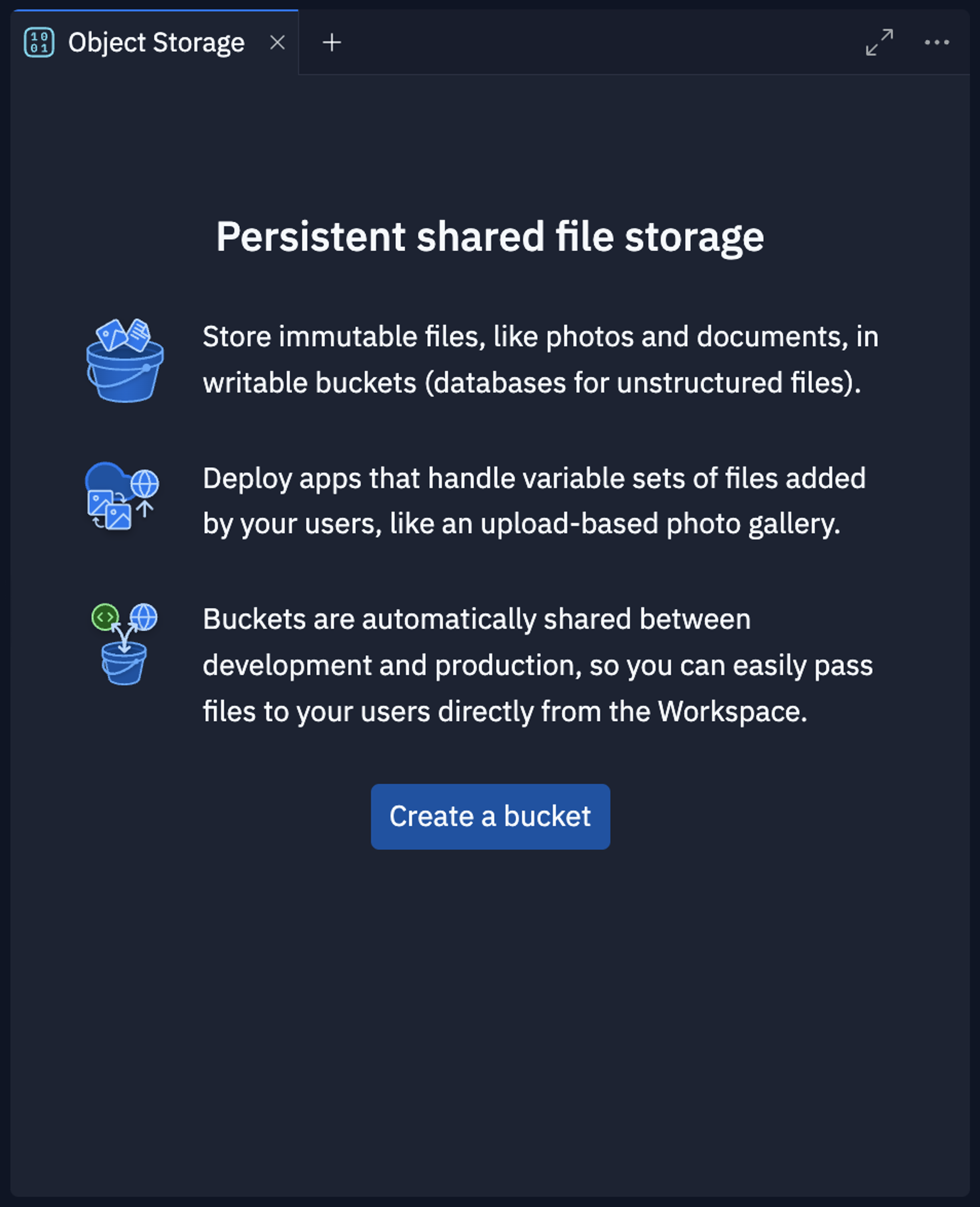
Once you’ve created a Bucket, you’ll see a view of all files in that bucket. Click “Upload File” to add your first object to the Bucket. The file will show up in your Bucket as soon as the upload completes. You can also download and delete your files from the same view.
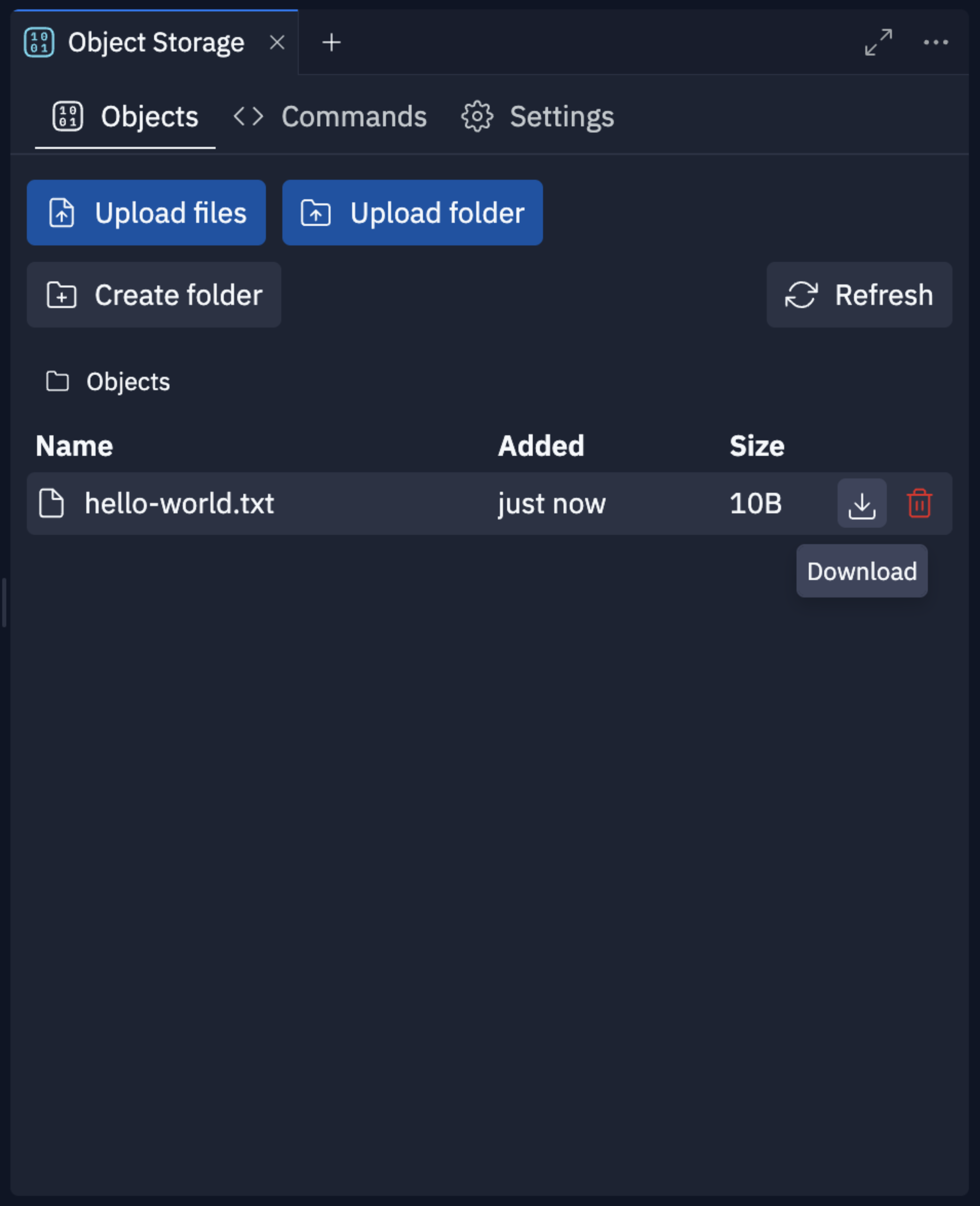
Now you’re up and running on Object Storage! To monitor how your bucket is getting used by your application (storage consumed, reads and writes per second), check out the “Resource usage” section on the Usage screen.
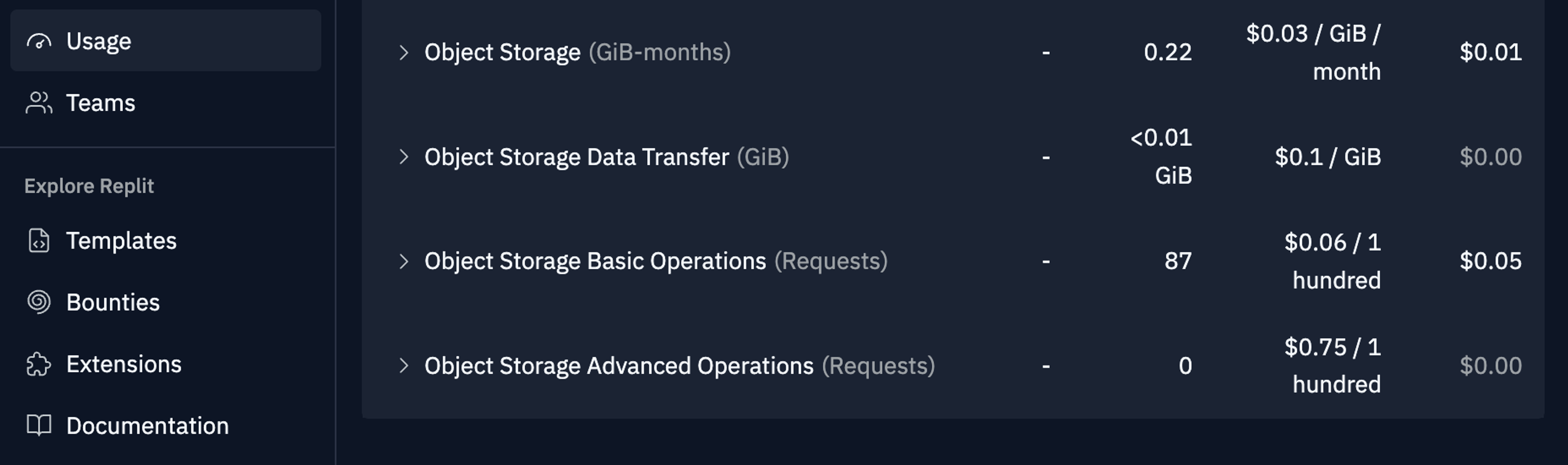
Introducing Replit Object Storage libraries
Python and Typescript users can now use the Replit Object Storage library to read and write files from Object Storage with zero configuration. If you’re using another language, we recommend using the GCP Client Libraries (see the next section, below).
Read and writing files in Python is as simple as:
from replit.object_storage import Client
client = Client()
contents = client.download_as_text("mark.txt")
client.upload_from_text("mark_copy.txt", contents)
Files will be accessible from the Repl where they were created. For example, a file written by your application by hitting the “Run” command in the workspace will also be accessible when your application runs in a Deployment. The same file won’t be accessible in other Repls.
To install the Replit Object Storage library in your application, open the Object Storage pane in the workspace and select the “Commands” view. Here you’ll find both a quick reference on library usage in your Repl’s language and “Install”, a one-click setup button that will run the installation.
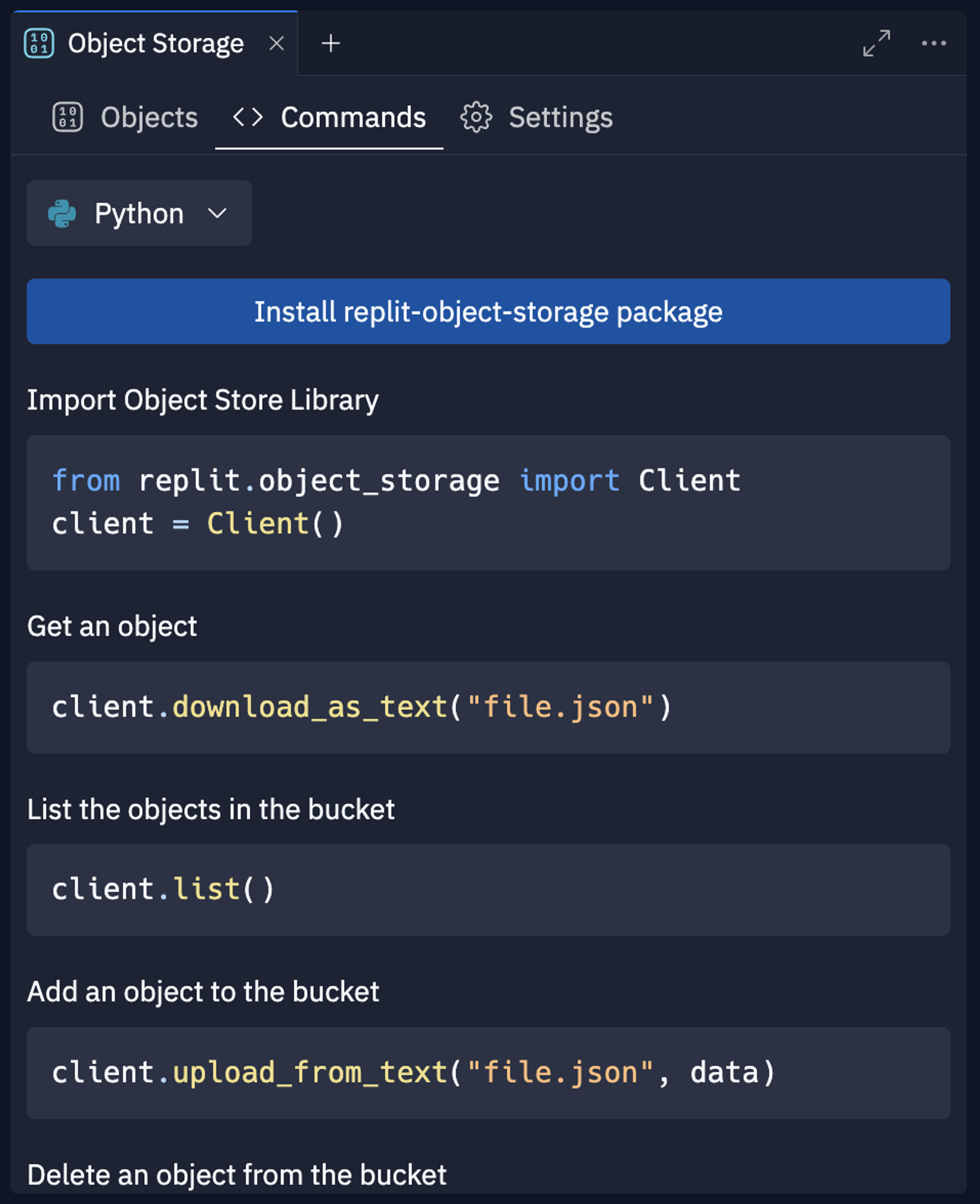
Leveraging Google Cloud Storage
One of the primary technologies behind Replit Object Storage is Google Cloud Storage (GCS). There are several reasons why Replit partners with Google:
- It is designed for effectively limitless storage with 99.999999999% (11 9s) durability and fast upload/download performance.
- It has an intuitive API which aligns with the experience we want to provide.
- Replit runs both Repls and Deployments on Google Cloud Platform (GCP), meaning we can reduce latency and minimize data ingress / egress costs (and reduce your costs!) by staying in the same network and region.
Replit provides you with direct access to GCP APIs. This means users will be able to directly use GCP Client Libraries in any language (at time of writing, this is Go, Java, Node.js, Python, Ruby, PHP, and C++) or via REST API. GCP Client Libraries work out-of-the-box with minimal configuration; many existing applications or third-party libraries that work with GCS will work with Replit Object Storage out-of-the-box.
Replit handles authentication and integrates with Google Application Default Credentials to provide no-code setup. For more information on how to set this up, visit the “Commands” tab in the Object Storage pane. We use the unique, verifiable identity of Repls to scope access to exactly the Object Storage resources that have been configured (one of our goals is to avoid complex IAM configuration).
In addition to broad language support, users can use advanced features of GCS such as preconditions and multipart or resumable uploads at launch by using official GCS libraries.
Pricing
See the pricing page for more information.